YS's develop story
[Spring] request시 notnull값 controllerAdvice로 처리해서 response보내기 본문
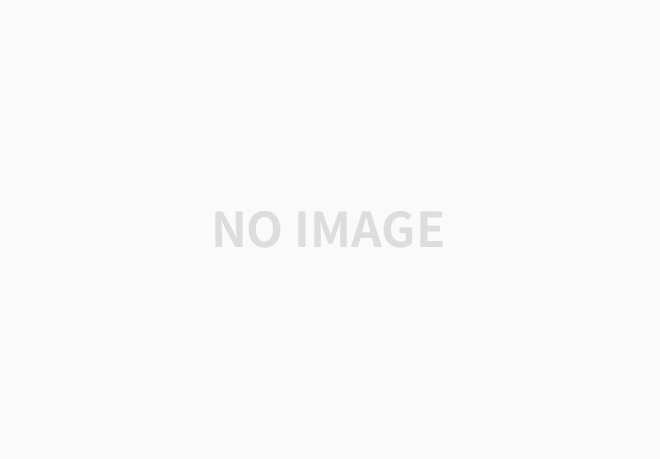
public record CreateReservationRequest(
@NotNull(message = "startDate cannot be null")
LocalDate startDate,
@NotNull(message = "endDate cannot be null")
LocalDate endDate,
@NotNull(message = "numberOfPerson cannot be null")
Integer numberOfPerson
) {
public Reservations toEntity(
User user, AccommodationRooms rooms, boolean paymentCompleted) {
return Reservations.builder()
.user(user)
.rooms(rooms)
.startDate(startDate)
.endDate(endDate)
.numberOfPerson(numberOfPerson)
.paymentCompleted(paymentCompleted)
.build();
}
}
@NotNull 어노테이션을 사용하면 해당 필드에 null 값이 들어오지 않도록 강제할 수 있습니다.
위와 같은 코드에서 request로 notnull값에 null이 들어오는 경우 Spring은 자동으로
MethodArgumentNotValidException을 던지게 됩니다.
하지만 이 예외는 핸들링되지 않기 때문에 직접 핸들링해서
알맞은 형식으로 response를 보내 주어야 합니다. 그렇게 해야 프론트개발자 입장에서 처리할 수 있습니다.
@ControllerAdvice 에서 아래와 같이 MethodArgumentNotValidException을 핸들링할 수 있습니다.
import org.springframework.validation.BindingResult;
import org.springframework.validation.FieldError;
import org.springframework.web.bind.MethodArgumentNotValidException;
@RestControllerAdvice
public class GlobalExceptionRestAdvice {
//notnull값을 넣지 않은 경우 에러 핸들링
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<ResponseDTO<Object>> handleValidationExceptions(
MethodArgumentNotValidException e) {
BindingResult bindingResult = e.getBindingResult();
//Notnull어노테이션에 작성한 에러메시지가 뜨도록 메시지 바인딩
Map<String, String> fieldErrors = bindingResult.getFieldErrors()
.stream()
.collect(Collectors.toMap(FieldError::getField, FieldError::getDefaultMessage));
log.error(e.getMessage(), e);
return ResponseEntity
.status(HttpStatus.BAD_REQUEST)
.body(
ResponseDTO.res(HttpStatus.BAD_REQUEST, fieldErrors));
}
}
실제로 null일 수 없는 값을 null로 하여 요청하면 커스텀 핸들러가 에러를 처리해서 response를 보내게 됩니다.
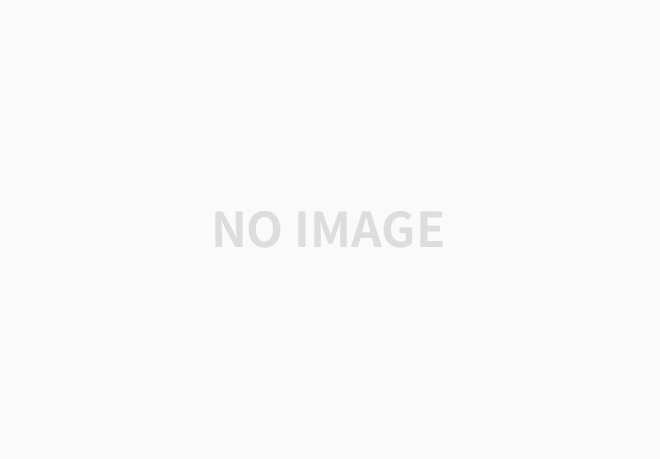
'Spring' 카테고리의 다른 글
spring jpa에 Querydsl 적용하기 [spring 3.1.5, java 17] (0) | 2023.12.06 |
---|---|
springboot certbot으로 ssl인증서 받아서 https로 배포하기 (1) | 2023.11.30 |
GCP 에서 springboot 프로젝트 docker로 배포하기 (1) | 2023.11.25 |
Jpa @CreatedDate, @LastModifiedDate 어노테이션이 적용 (0) | 2023.11.22 |
Spring, Docker에서 MySQL 데이터베이스 컨테이너 설정하기 (0) | 2023.11.19 |